Golang is a general purpose programming language with advanced features and a clean syntax. Because of its wide availability on a variety of platforms, its robust well-documented common library, and its focus on good software engineering principles. It is an ideal language to learn as your first programming language.
It is the most exciting new mainstream language to appear in at least 15 years and is the first such language that is aimed squarely at 21st century computers and their programmers. It is designed to scale efficiently so that it can be used to build very big applications and to compile even a large program in mere seconds on a single computer.
The lightning-fast compilation speed is made possible to a small extent because the language is easy to parse, but mostly because of its dependency management. It has an open development model and many developers from around the world contribute to it, with some so trusted and respected that they have the same commit privileges as the Googlers.
Since Go programs are so fast to build, it is practical to use them in situations where scripting languages are normally used.
This career guide at a glance:
- Step 1: Learn the basics
- Step 2: Master core features (functions, pointers, structs, interfaces)
- Step 3: Handle program errors smoothly
- Step 4: Promote code reuse with packages
- Step 5: Write powerful concurrent software with Goroutines and channels
- Step 6: Test and benchmark Golang code
- Step 7: Learn how to create command line programs
- Step 8: Learn how to create JSON REST APIs
- Step 9: Study the Masters , Make Something and Team Up
- Step 10: Conclusion and what next to learn
Learn the basics
Work with types, variables, functions, and control structures
Golang has a concept of types that is either explicitly declared by a programmer or is inferred by the compiler.
Languages are said to be “strongly typed” if a compiler throws an error when types are used incorrectly.
Languages are said to be “dynamically typed” (also known as “loosely typed” and “weakly typed”) if a runtime converts types from one type to another in order to execute the program, or if the compiler does not enforce the type system.
Statically typed language advantages:
- better performance than dynamically typed languages
- bugs can often be caught by a compiler
- code editors can offer code completion and other tooling
- better data integrity
Dynamically typed language advantages:
- it is often faster to write software using a dynamically typed language
- there is no need to wait for a compiler to execute code
- dynamically typed languages are generally less rigid, and some argue that they are more open to change
- some argue there is a shorter learning curve
With a basic understanding of types, you can now explore some fundamental data types implemented by Go, like booleans and numeric types, as well as checking the type of a variable and converting between types.
Variables are a fundamental part of writing computer programs. A variable is simply a reference to a value, and is one of the foundations of being able to construct programming logic.
In Go, declaring a variable can be achieved in a number of ways. Now after understanding of types you’ll have to Learn about:
- what is a variable?
- declaring shorthand variables
- understanding variables and zero values
- writing a short variable declaration
- which style of variable declaration is best
- using variable scope
- using pointers
- declaring variables with constants
- and more
Functions represent another core construct of not just Golang but programming in general.
Now you learn about using functions in Go, beginning with a simple function that returns a single result.
You will see how Golang can return multiple results, accept a varying number of arguments, and how it displays some features that make it look somewhat like a functional programming language.
You have to go through various topics like:
- defining variadic functions
- using named return values
- using recursive functions
- passing off functions as values
- and more
About control structures and how the flow of execution of code is determined. This allows you to construct programs that respond to data in different ways. To support this, you learn about the if statement and how to build up logic using comparison, methodical, and logical operators. You will understand how to use loops and to iterate over blocks of code multiple times. Finally, you are introduced to defer that allows a function to be executed after another function has finished.
Make the most of Go’s arrays, slices, and maps
Now you are introduced to some of the fundamental building blocks of Go. You learn how to initialize and assign values to elements in an array. You also learn about the difference between arrays and slices in Go. You learn how to add and remove elements from a slice before being introduced to maps.
Arrays are an important part of Go, but it is much more common to see and use slices in Go. A slice is defined as a “contiguous segment of an underlying array and provides access to a numbered sequence of elements from that array.” As such, slices provide access to parts of an array in sequential order. Why then do slices exist? Why not just use arrays? Using arrays in Golang has some limitations. Slices offer more flexibility than arrays in that it is possible to add, remove, and copy elements from a slice. Slices can be considered a lightweight wrapper around arrays that preserves the integrity of the array type but makes arrays easier to work with.
Arrays and slices are a collection of elements that may be accessed by an index value. A map is an unordered group of elements that is accessed by a key rather than an index value. While the word array is common to most programming languages, a map can often be known as an associative array, dictionary, or hash if you have experience in other programming languages. Maps can be very efficient in looking up pieces of information, as the data can be retrieved directly through the key. In simple terms, maps can be considered a collection of keys and values. An empty map can be initialized in a single line of code.
Master core features (functions, pointers, structs, interfaces)
As its name suggests, a struct is a structure of data elements that is a useful programming construct. You should introduce yourself to structs and the various ways to initialize them. You will understand how default values are assigned and how to assign a custom default value to data fields. You will see how to compare structs and learn about exported values. Finally, you will learn the subtle difference between using pointers and values in relation to underlying memory.
Sometimes, data structures need more than one level of elements. Although you might choose to use a data type, like a slice, there are times when a data structure is more complex. Nesting one struct within another can be a useful way to model more complex structures.
When working with structs, it is important to understand the difference between pointer and value references. A pointer holds the memory address of a value, meaning that when it is referenced, it is possible to read or write the value that has been stored. When a struct is initialized, memory is allocated for the data elements and their default values in the struct. A pointer to this memory is returned, and this is assigned to a variable name. The difference between pointers and values is a subtle one, but choosing whether to use a pointer or a value is simple. If you need to modify (or mutate) the original initialization of a struct, use a pointer. If you need to operate on a struct, but do not want to modify the original initialization of a struct, use a value.
You learned about structs and understood that structs are a way to create data structures. You understood that it is possible to access data within structs using dot notation. Once operations get beyond being trivial, however, it can become more complex to reason about and to manipulate data. Golang provides another way to operate on data through function. In this, you are introduced to function and how to create and use method sets that are associated with a data type. You are then introduced to interfaces, a way to describe method sets that offer powerful modularity.
An interface specifies a method set and is a powerful way to introduce modularity in Go. You can think of interfaces as a blueprint for a method set, in that an interface describes all the function in a set but does not implement them. Interfaces are powerful since they act as a specification for a method set, meaning that implementations can be swapped, providing they conform to the expectations of an interface
Handle program errors smoothly
It is a fact of life that software will contain errors and scenarios that are not accounted for. Many languages choose to throw exceptions when errors occur that must be caught or trapped. Golang offers an interesting approach to errors in that they are a type in the language. This means that they may be passed around functions and function. During this section, you will be introduced to error handling in Golang and how you can use error handling to your advantage.
Error handling is an important part of programming robust, reliable, and maintainable code. If you are writing code for yourself, it can help account for unexpected scenarios. If you are writing libraries or packages that others will use, handling errors is part of creating robust, reliable, and trustworthy code.
When calling a method or function that could fail, one of Go’s conventions is to return an error type as its final value. This means that generally no exception will be thrown within a function if something goes wrong. Instead, it is up to the caller to decide what to do with the error.
Promote code reuse with packages
Golang was designed to be a language that encourages good software engineering practices. An important part of high quality software is code reuse – embodied in the principle “Don't Repeat Yourself.” Golang also provides another mechanism for code reuse: packages.
Nearly every program we've seen so far included this line import "fmt"
. Fmt
is the name of a package that includes a variety of functions related to formatting and output to the screen. Bundling code in this way serves 3 purposes:
- it reduces the chance of having overlapping names. This keeps our function names short and succinct
- it organizes code so that it’s easier to find code you want to reuse.
- it speeds up the compiler by only requiring recompilation of smaller chunks of a program. Although we use the package fmt, we don't have to recompile it every time we change our program.
A module is a collection of Golang packages stored in a file tree with a go.mod file at its root. The go.mod file defines the module’s module path, which is also the import path used for the root directory, and its dependency requirements, which are the other modules needed for a successful build. Each dependency requirement is written as a module path and a specific semantic version.
The go command enables the use of modules when the current directory or any parent directory has a go.mod, provided the directory is outside $GOPATH/src. (Inside $GOPATH/src, for compatibility, the go command still runs in the old GOPATH mode, even if a go.mod is found. See the go command documentation for details.). A sequence of common operations that arise when developing Golang code with modules:
- creating a new module
- adding a dependency
- upgrading dependencies
- adding a dependency on a new major version
- upgrading a dependency to a new major version
- removing unused dependencies
The Golang standard library includes a large number of packages that between them provide a wide range of functionality. The overview provided here is highly selective and very brief:
- Archive and Compression Packages
- Bytes and String-Related Packages
- Collection Packages
- File, Operating System, and Related Packages
- File Format-Related Packages
- Graphics-Related Packages
- Mathematics Packages
- Miscellaneous Packages
- Networking Packages
- The Reflect Package
Write powerful concurrent software with Goroutines and channels
In most simple computer programs, things happen sequentially and are executed in the order they appear. In a simplistic way, think of the lines of code in a script. Lines are executed in the order they appear. Until one line has finished executing, the next line will not be executed. For many programs this is the desired behavior, and allows a programmer to reason about the logic of a script safe in the knowledge that one line will not be executed until the next one has finished.
In this, now you will be introduced to the idea of concurrency and Goroutines. You will understand the difference between sequential execution and concurrent execution. You will see how Goroutines can be one way to deal with a network latency. You will be introduced to the difference between concurrency and parallelism and see how Goroutines make programs run faster. Goroutines are one of Go’s most elegant features, so if at any stage you feel this looks complicated, read on. Golang solves it all with one keyword!
As you have introduced to Goroutines as a way to handle concurrent operations. You saw how it is possible to program tasks to run concurrently and handle responses when they return. You also saw that it was necessary to create timers to manage Goroutines. In future you will see Channels, a way to manage communication between Goroutines. When combined, Channels and Goroutines offer a carefully curated environment in which to develop concurrent software.
Test and benchmark Golang code
Writing tests for our code is a good way to ensure quality and improve reliability. Golang includes a special program that makes writing tests easier. Testing software programs is perhaps the most important thing a software developer can do. By testing the expected functionality of code, a developer can have a good level of confidence that a program works. Furthermore, a developer can run tests each time a code change occurs, and have confidence that bugs and regressions have not been introduced. Testing software also allows a software engineer to declare the expected way a program should work.
Package testing provides support for automated testing of Golang packages. It is intended to be used in concert with the "go test" command, which automates execution of any function of the form. https://golang.org/pkg/testing/
Often, software tests are derived from a User Story or Specification that outlines how a feature should work. For example, if a User Story expresses that a function should take two numbers, add them together, and return the result, a test can easily be written to test this. Some projects also mandate that new code has an accompanying test. Well-written tests can act as documentation. Since a test describes the expected way a program should run, developers joining a project can often read tests as a way to understand how a program operates. Several types of tests are commonly used.
Unit Tests : Unit tests cover small parts of a code base and test these in isolation. Often, this might be a single function, and the inputs and outputs of a function are tested. A typical unit test might say, “If I give function x these values, I should expect this value to be returned.”
Functional Tests: Integration tests typically test how the various parts of an application work together. If unit tests verify the smallest parts of a program, integration tests look at how the components of an application work together.
Integration Tests: Functional tests are often known as end-to-end tests and outside-in tests. These tests verify that software works as expected from an end-user perspective. They assess how a program works from the outside without being concerned with how the software works internally.
For users of software, functional tests are perhaps the most important tests that can be run. Examples of functional tests include:
- testing that a command line tool responds to certain inputs with certain outputs
- running automated tests on a web page
- running outside-in tests against an API to check response codes and headers
Golang also offers a powerful benchmarking framework that allows discussions over the most performant way of doing something to be resolved with a benchmark. The testing package includes a powerful benchmarking framework that allows a function to be run repeatedly and a benchmark to be applied. The number of times a function is run is not set, but is adjusted by the benchmarking framework to have a reliable data set. At the end of the benchmark, a report is given on the number of operations that were complete per nanosecond. Benchmark tests start with the Benchmark keyword and take the B type as an argument that provides functions to benchmark code.
Learn how to create command line programs
A command-line program (sometimes also called a command-line utility or tool) is a program designed to be run from a terminal. In this , you will learn how to create a command-line program with Go. You will understand how to parse arguments and move on to create subcommands. Before graphical user interfaces (GUI), it was normal to interact with a computer via the command line. Today, command-line utilities continue to be a popular and practical way for programmers and systems administrators to interact with an underlying operating system. A programmer might want to create a command-line program to:
- Create a script that can be run automatically on a regular basis.
- Create a script to interact with files on an operating system.
- Create a script to perform system maintenance tasks.
- Avoid the unnecessary overhead of designing a graphical interface.
A command-line program normally performs an operation like managing files in a directory or taking some input data and returning some output data. A good example of this is the sort command that is present on 228Windows, Linux, and macOS
Learn how to create JSON REST APIs
During this part, you learn how to work with JSON in Go. You will learn how to encode and decode JSON and understand some of the differences between JavaScript and Golang data types. You will understand how to use struct tags to have finer control over JSON and see how to fetch JSON from remote APIs using HTTP.
In recent years, many excellent JSON APIs have been created on the Internet. It is now possible to have access to a wealth of data on almost any subject using the Internet as a data exchange platform. Instead of connecting directly to a database, APIs allow programmers to request data in a range of formats and to use the data. So basically ,if you are writing any form of web application, then you are most likely interfacing with 1 or more REST APIs in order to populate the dynamic parts of your application and to perform tasks such as updating or deleting data within a database. You will know how to create your own REST-ful APIs in Golang that can handle all aspects of. You will know how to create REST endpoints within your project that can handle POST, GET, PUT and DELETE HTTP requests
Application developers have used many of these APIs to create new and interesting products and services. There are many Dark Sky clients for Android, for example, and there is an emerging business model of being a data provider and allowing customers to consume the data however they like. Golang is an excellent language for creating clients and servers that send and receive JSON. The standard library provides support for decoding and encoding data to JSON format through the encoding/json package.
Golang offers strong support for creating web servers that can serve web pages, web services, and files. During this section, you will learn how to create web servers that can respond to different routes, different types of requests, and different content types. By taking advantage of Go’s approach to concurrency, writing web servers in Golang is a great option. The net/http standard library package provides multiple methods for creating HTTP servers, and comes with a basic router. With curl installed, you can use it for developing and debugging web servers. Rather than using a browser, you can use curl to send a variety of requests to a web server and to examine the response. To make a request to the Hello World web server, open a terminal and run the server.
On macOS or Linux, open another terminal tab or window. On Windows, switch to Git Bash. Run the following command. The -is options mean that headers are printed and that some unwanted output is ignored.
curl -is http://localhost:8000
The curl guide to http request https://flaviocopes.com/http-curl/ . If the command was successful, you will see a response from the web server that includes the headers and the response body.
Get productive quickly with Golang development tools and web servers. Efficiently move Golang code into production. Hypertext Transfer Protocol (HTTP) is the network protocol used to send and receive resources on the Internet. Among other things, it’s used to transmit images, HTML documents, and JSON. In this , you learn how to create HTTP clients using Go. You will learn how to make different types of requests and how to debug your program during development.
Study the Masters , Make Something and Team Up
Part of becoming a good artist or writer is studying the works of the masters. It's no different with programming. One of the best ways to become a skilled programmer is to study the source code produced by others. Golang is well suited to this task since the source code for the entire project is freely available. For example we might take a look at the source code to the io/ioutil library available at: http://golang.org/src/pkg/io/ioutil/ioutil.go
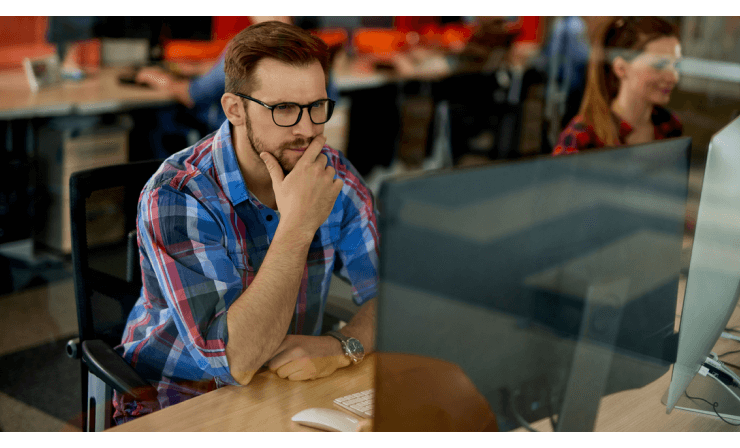
Read the code slowly and deliberately. Try to understand every line and read the supplied comments. For example in the ReadFile method there's a comment that says this:
- It's a good but not certain bet that FileInfo
- will tell us exactly how much to read, so
- let's try it but be prepared for the answer
- to be wrong.
This method probably started out simpler than what it became so this is a great example of how programs can evolve after testing and why it's important to supply comments with those changes. All of the source code for all of the packages is available at: http://golang.org/src/pkg/
One of the best ways to hone your skills is to practice coding. There are a lot of ways to do this: You could work on challenging programming problems from sites like Project Euler (http://projecteuler.net/) or try your hand at a larger project. Perhaps try to implement a web server or write a simple game. https://golang.org/project/
Most real-world software projects are done in teams, therefore learning how to work in a team is crucial. If you can, find a friend ,maybe a classmate and team up on a project. Learn how to divide a project into pieces you can both work on simultaneously. Another option is to work on an open source project. Find a 3rd party library, write some code (perhaps fix a bug), and submit it to the maintainer. Golang has a growing community which can be reached via the mailing list (http://groups.google.com/group/golang-nuts).
Conclusion and what next to learn
The best place to go for the latest information on Golang is golang.org; this web site has the current release’s documentation, the (very readable) language specification, the Golang Dashboard, blogs, videos, and numerous other supporting documents. We now have all the information we need to write most Go programs. But it would be dangerous to conclude that therefore we are competent programmers. Programming is as much a craft as it is just having knowledge.
These topics will provide you some advanced knowledge and they are the next right thing that you want to learn:
- Working with Websockets and Socket.IO
- Protocol Buffer
- WebAssembly Tutorial - Building a Calculator Tutorial
- Oauth2
- Face Recognition
- Encryption and Decryption using AES
- Building a Solid Continuous Integration Pipeline with TravisCI for Your Golang Projects
- Secure Coding - Input Validation
- Writing a Frontend Web Framework with WebAssembly And Go
- Securing Your REST APIs With JWTs
- https://tutorialedge.net/course/golang/
- https://www.golangprograms.com/advance-programs.html
Books
If you want to master this language it’s always a good choice to read books and these books makes few assumptions about programming or computer science experience, but it is helpful to have some basic understanding of programming.
As Golang is primarily run from the terminal, it is helpful to understand what a terminal is and how to run basic commands. Finally, because Golang is often used for systems and network programming, it is helpful to understand a little of how the Internet works, although this is not essential.
These books start with the basics of Go, including setting up your environment and running your first Go program. You are then introduced to some of the fundamentals of the language, including strings, functions, structs, and methods. You will understand how to use Goroutines and Channels, a unique feature of Golang that abstracts much of the difficulty of concurrent programming. You will be introduced to how to debug and test Golang code, and will be introduced to some techniques that will help you to understand how to write idiomatic Golang code. You will then understand how to write basic command-line programs, HTTP servers, and HTTP clients; how to work with JSON; and how to work with files. After learning about Regular Expressions and how to work with time, these books conclude by explaining how to deploy Golang applications to production.